Flash CS3 Drawing API, ActionScript 3, Shape Class
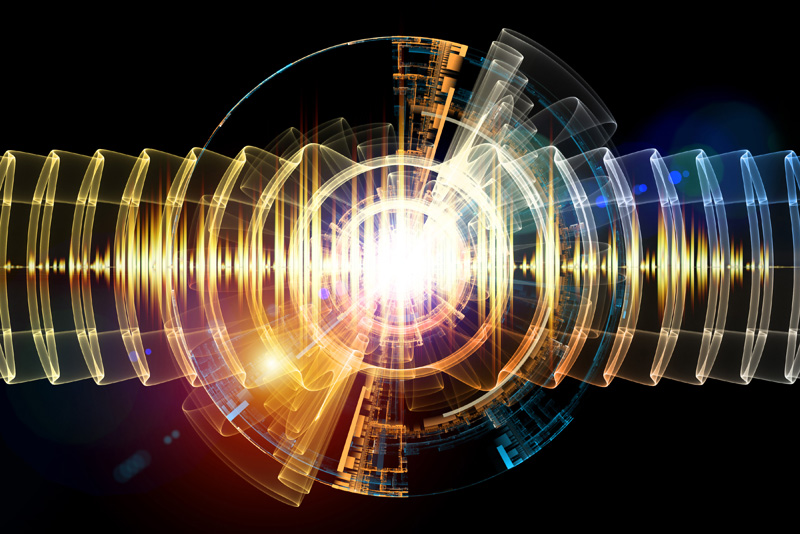
One of my favorite things to do in Flash is to draw onto the Stage. But I am not talking about drawing with the Flash drawing tools such as the Rectangle or Oval tool. In this tutorial, we will learn the basics for drawing with ActionScript 3 and the Drawing API.
I hesitate to talk too much about OOP programming and classes in my beginner's tutorials because it just makes things more complicated. But this tutorial is an exception. It is perfect for introducting the basic concept of OOP classes. An OOP class in ActionScript is just a prebuilt bunch of information, called properties and methods. This prebuilt information saves the programmer a lot of time when coding ActionScript because it is not necessary to rewrite this information in every program which calls for the use a specific class.
In this tutorial, we will be using the Shape class, its predefined Graphics property and several of its methods to draw a simple circle on the stage.
Graphics property
lineStyle method
beginFill method
drawCircle method
endFill() method
Our first step is to create an Actions layer on the Timeline, click on Frame 1 and open the Actions panel (Window - Actions). Our first line of code will create a new variable that will hold the circle.
var myCircle:Shape = new Shape();
In the above code, we are creating a new variable and naming it "myCircle". We are setting the data type for this new variable to Shape. On the other side, we are using the new keyword to create a new instance of the Shape class.
If you have used the Flash drawing tools, you know that a shape has both a Stroke and Fill. When using the Shape class to draw, you also have a Stroke and Fill. In the next few lines of code, we will define the Stroke and Fill for our myCircle.
myCircle.graphics.lineStyle(2, 0x000000);
In the code above, we are setting the Stroke values with the lineStyle method of the Graphics property. We will set the value of the width of the Stroke in pixels and the color of the Stroke with a hexadecimal value. Above, we have set the Stroke for our circle to a width of 2 pixels and a color of black.
Next, we will fill the circle. Again we will use the Graphics property and its beginFill method. The two values that we can control for the Fill of the circle are the color and alpha. It is required that you set a value for the color and if you don't, Flash will get very upset. But, setting the alpha value is optional. Let's set the Fill for our circle to red. Again we use the hexadecimal.
myCircle.graphics.beginFill(0xff0000);
OK, now we need to tell Flash where to draw the circle on the stage. As you would expect, you need to set a value for the X and Y axes and the radius of the circle. We will do this with the drawCircle method which is part of the Graphics property.
myCircle.graphics.drawCircle(100, 200, 50);
In this line of code, we are telling Flash to draw our circle 100 pixels from the upper left corner of the stage along the X axis and 200 pixels along the Y axis. Our circle will have a radius of 50. When you set these values, take care of the order in which you list them in the code. Your first value will be for the X axis and the second value is for the Y axis. The third is for the radius. If you give Flash these values out of order, you will get some unexpected results.
Finally, we need to add our circle as a child display object to the display list .
addChild(myCircle);
Oh, I left our one line of code! Although our code will work as is, we have left out the endFill() method which will "close" the drawing process. This is not really necessary because the endFill() is called automatically. But it is good practice to add it to your code just before the addChild().
myCircle.graphics.endFill();
Here is the complete code.
Copyright 2018 Adobe Systems Incorporated. All rights reserved. Adobe product screen shot(s) reprinted with permission from Adobe Systems Incorporated. Adobe, Photoshop, Photoshop Album, Photoshop Elements, Illustrator, InDesign, GoLive, Acrobat, Cue, Premiere Pro, Premiere Elements, Bridge, After Effects, InCopy, Dreamweaver, Flash, ActionScript, Fireworks, Contribute, Captivate, Flash Catalyst and Flash Paper is/are either [a] registered trademark[s] or a trademark[s] of Adobe Systems Incorporated in the United States and/or other countries.
I hesitate to talk too much about OOP programming and classes in my beginner's tutorials because it just makes things more complicated. But this tutorial is an exception. It is perfect for introducting the basic concept of OOP classes. An OOP class in ActionScript is just a prebuilt bunch of information, called properties and methods. This prebuilt information saves the programmer a lot of time when coding ActionScript because it is not necessary to rewrite this information in every program which calls for the use a specific class.
In this tutorial, we will be using the Shape class, its predefined Graphics property and several of its methods to draw a simple circle on the stage.
Graphics property
lineStyle method
beginFill method
drawCircle method
endFill() method
Our first step is to create an Actions layer on the Timeline, click on Frame 1 and open the Actions panel (Window - Actions). Our first line of code will create a new variable that will hold the circle.
var myCircle:Shape = new Shape();
In the above code, we are creating a new variable and naming it "myCircle". We are setting the data type for this new variable to Shape. On the other side, we are using the new keyword to create a new instance of the Shape class.
If you have used the Flash drawing tools, you know that a shape has both a Stroke and Fill. When using the Shape class to draw, you also have a Stroke and Fill. In the next few lines of code, we will define the Stroke and Fill for our myCircle.
myCircle.graphics.lineStyle(2, 0x000000);
In the code above, we are setting the Stroke values with the lineStyle method of the Graphics property. We will set the value of the width of the Stroke in pixels and the color of the Stroke with a hexadecimal value. Above, we have set the Stroke for our circle to a width of 2 pixels and a color of black.
Next, we will fill the circle. Again we will use the Graphics property and its beginFill method. The two values that we can control for the Fill of the circle are the color and alpha. It is required that you set a value for the color and if you don't, Flash will get very upset. But, setting the alpha value is optional. Let's set the Fill for our circle to red. Again we use the hexadecimal.
myCircle.graphics.beginFill(0xff0000);
OK, now we need to tell Flash where to draw the circle on the stage. As you would expect, you need to set a value for the X and Y axes and the radius of the circle. We will do this with the drawCircle method which is part of the Graphics property.
myCircle.graphics.drawCircle(100, 200, 50);
In this line of code, we are telling Flash to draw our circle 100 pixels from the upper left corner of the stage along the X axis and 200 pixels along the Y axis. Our circle will have a radius of 50. When you set these values, take care of the order in which you list them in the code. Your first value will be for the X axis and the second value is for the Y axis. The third is for the radius. If you give Flash these values out of order, you will get some unexpected results.
Finally, we need to add our circle as a child display object to the display list .
addChild(myCircle);
Oh, I left our one line of code! Although our code will work as is, we have left out the endFill() method which will "close" the drawing process. This is not really necessary because the endFill() is called automatically. But it is good practice to add it to your code just before the addChild().
myCircle.graphics.endFill();
Here is the complete code.
Copyright 2018 Adobe Systems Incorporated. All rights reserved. Adobe product screen shot(s) reprinted with permission from Adobe Systems Incorporated. Adobe, Photoshop, Photoshop Album, Photoshop Elements, Illustrator, InDesign, GoLive, Acrobat, Cue, Premiere Pro, Premiere Elements, Bridge, After Effects, InCopy, Dreamweaver, Flash, ActionScript, Fireworks, Contribute, Captivate, Flash Catalyst and Flash Paper is/are either [a] registered trademark[s] or a trademark[s] of Adobe Systems Incorporated in the United States and/or other countries.

Related Articles
Editor's Picks Articles
Top Ten Articles
Previous Features
Site Map
Follow @ArtAnimationTut
Tweet
Content copyright © 2023 by Diane Cipollo. All rights reserved.
This content was written by Diane Cipollo. If you wish to use this content in any manner, you need written permission. Contact Diane Cipollo for details.